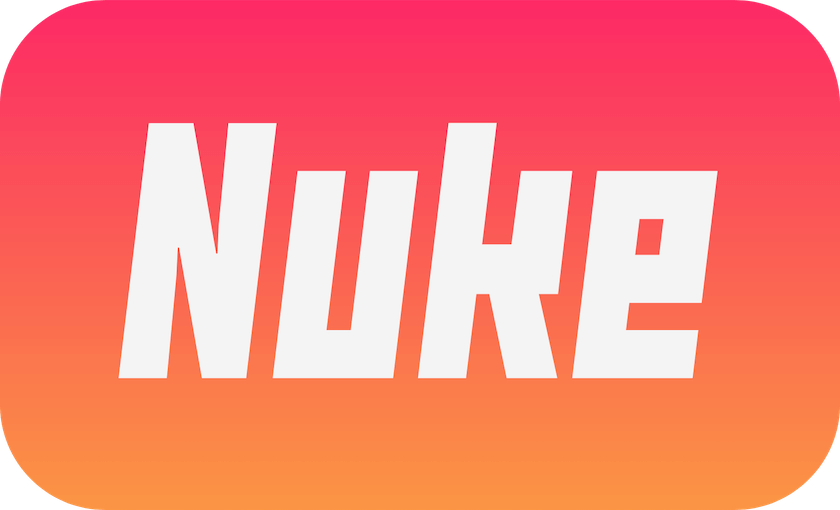
A powerful image loading and caching system
Fast LRU memory and disk cache · Background decompression · Image processing · HTTP range requests · Task coalescing · Prioritization · Low data mode · Prefetching · Progressive JPEG · WebP, GIF, HEIF · SwiftUI · Async/Await · Combine
Modern API
Nuke works on all Apple platforms and is frequently updated to support all new language and platform features, for example, Async/Await and SwiftUI.
// Load images directly using Async/Await
func loadImage() async throws {
let response = try await pipeline.image(for: url, delegate: self)
}
// Use UI components provided by NukeUI mode
struct ContainerView: View {
var body: some View {
LazyImage(url: URL(string: "https://example.com/image.jpeg"))
}
}
Caching
Fast LRU memory cache, native HTTP disk cache, and custom aggressive LRU disk cache.
Integrations
Customize image pipeline using built-in Alamofire, Gifu, FLAnimatedImage, WebP plugins or create your own.
Progressive Rendering
Nuke supports progressive JPEG out of the box, and WebP via a plugin built by the community.
Prefetching
Automatically prefetch images ahead of time using table and collection view prefetching APIs.
Resumable Downloads
If the request is terminated (either because of failure or cancellation) and the image was partially loaded, the next load will resume where it left off (HTTP range requests).
Animated Images
Use either FLAnimatedImage or Gifu plugin to display animated GIFs with smooth scrolling performance and low memory footprint.
Note: GIF is not the most efficient format for transferring and displaying animated images. The current best practice is to use short videos instead and NukeUI components support video playback.
Performance
Nuke does as little work on the main thread as possible. It is at least 3.5x faster than some competitive frameworks. It uses multiple optimizations to achieve that: reducing allocations, reducing dynamic dispatch, CoW, and more.
Robustness Under Stress
Nuke keeps memory usage under control. It is fully asynchronous and uses dedicated queues with limited concurrent task count. Nuke will even automatically rate limit the requests if necessary.
Smooth Scrolling
Nuke is tuned to do very little work on the main thread which helps achieve buttery smooth scrolling performance in table and collection views.